Authentication
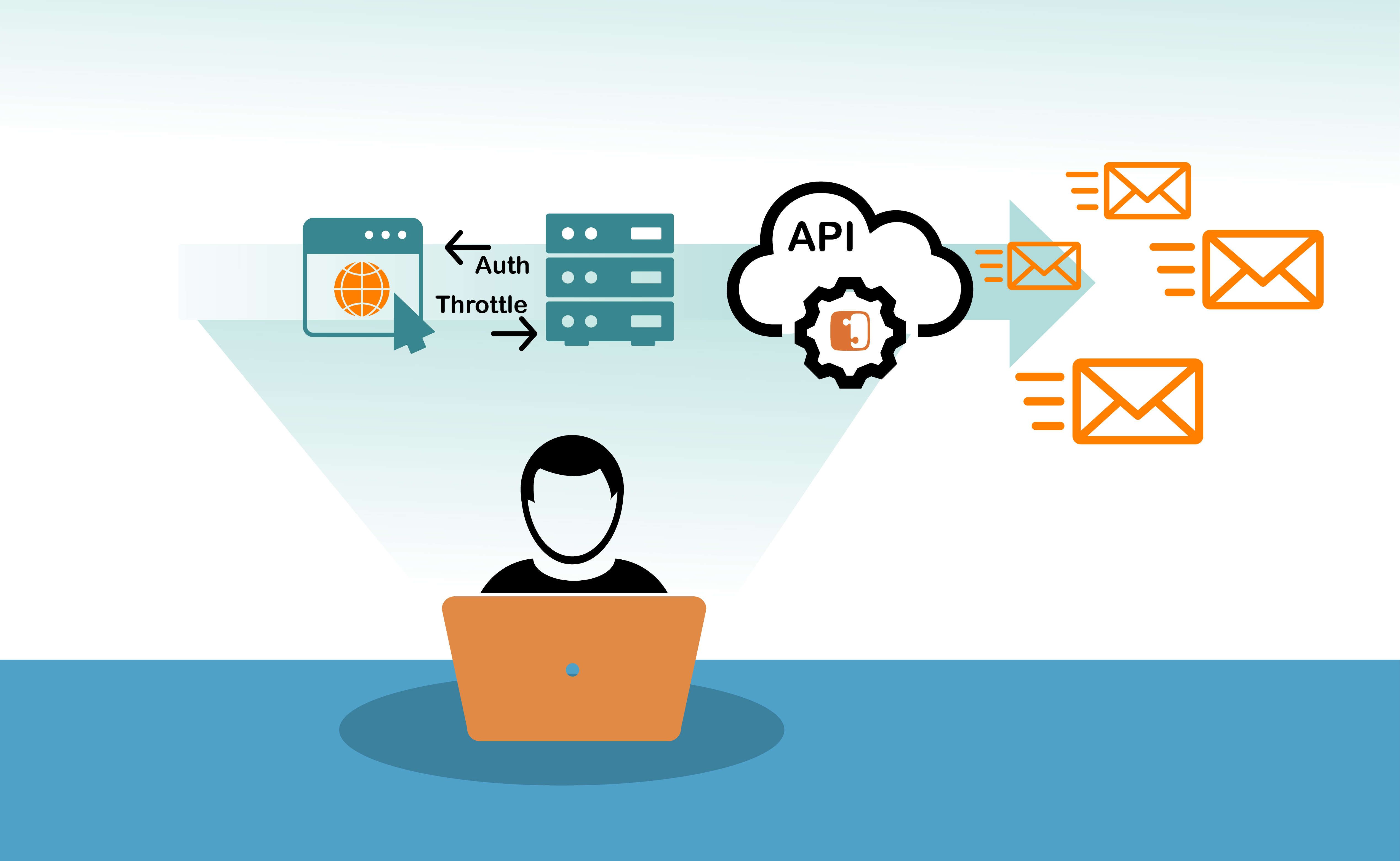
This article listing some important security measures that you need to check when you are designing, testing, and publishing your API.
Do not use Basic Auth to use standard authentication protocols (e.g. JWT, OAuth). Don’t make authentication, token generating, password storing these wheels, use standard ones.
Use Max Retry and automatic block function in login. Encrypt all sensitive data.
JWT (JSON Web Token)
Use random and complex keys (JWT Secret) to increase the difficulty of brute force cracking. Don’t extract data directly in the request body, encrypt the data (HS256 or RS256).
Keep the expiration time of the token as short as possible (TTL, RTTL). Do not store sensitive data in the JWT request body, it is hackable.
OAuth authorization or authentication protocol
Always verify the redirect_uri in the background, and only allow whitelisted URLs. Don’t add token every time you exchange tokens (response_type=token is not allowed).
Use the state parameter and fill in a random hash number to prevent cross-site request forgery (CSRF). Define the default scope and valid scope parameters for different applications.
Access
Limit traffic to prevent DDoS attacks and brute force attacks.
Use the HTTPS protocol on the server to prevent MITM attacks. Use HSTS protocol to prevent SSLStrip attacks.
Filter input
Use HTTP operation functions that match the operation, GET (read), POST (create), PUT (replace/update) and DELETE (delete record), and return 405 Method Not Allowed if the requested method is not applicable to the requested resource. The content-type field in the request header uses content validation to allow only supported formats (such as application/xml, application/json, etc.) and returns 406 Not Acceptable when the conditions are not met.
Verify that the content-type published data is the same as the one you received (such as application/x-www-form-urlencoded, multipart/form-data, application/json, etc.).
Verify user input to avoid common vulnerable flaws (such as XSS, SQL-injection, remote code execution, etc.).
Do not use any sensitive data (credentials, Passwords, security tokens, or API keys) in the URL, but use standard authentication request headers.
Use an API Gateway service to enable caching, access rate limiting (e.g. Quota, Spike Arrest, Concurrent Rate Limit), and dynamically deploy APIs resources.
Deal with
Check whether all terminals are after identity authentication to avoid a damaged authentication system. Avoid using unique resource id. Use /me/orders instead of /user/654321/orders Use UUID instead of self-increasing id.
If you need to parse XML files, make sure that entity parsing is turned off to avoid XXE attacks.
If you need to parse XML files, make sure that entity expansion is turned off to avoid the Billion Laughs/XML bomb implemented by exponential entity expansion attacks.
Use CDN in file upload.
If you need to process a lot of data, use Workers and Queues to respond quickly to avoid HTTP blocking. Don’t forget to turn off DEBUG mode.
Output
Send the X-Content-Type-Options: nosniff header.
Send the X-Frame-Options: deny header.
Send the Content-Security-Policy: default-src’none’ header.
Delete fingerprint head-X-Powered-By, Server, X-AspNet-Version, etc. Mandatory use of content-type in the response, if your type is application/json, then your content-type is application/json.
Don’t return sensitive data, such as credentials, Passwords, security tokens. Return the appropriate status code at the end of the operation. (e.g. 200 OK, 400 Bad Request, 401 Unauthorized, 405 Method Not Allowed, etc.).
Continuous integration and continuous deployment
Use unit tests and integration tests to audit your design and implementation. Introduce a code review process, don’t approve changes yourself.
Before pushing to the production environment, ensure that all components of the service are statically scanned with anti-virus software, including third-party libraries and other dependencies. Design a rollback plan for deployment.
Authority system (registration/registration/secondary verification/password reset)
Use HTTPS everywhere.
Use Bcrypt to store password hashes (there is no need to use salt-this is what Bcrypt does).
Destroy the session ID after logging out.
Destroy all active sessions after password reset.
OAuth2 authentication must include the state parameter.
After successfully logging in, you cannot directly redirect to the open path (verification is required, otherwise phishing attacks are prone to occur).
When parsing user registration/login input, filter javascript://, data:// and other CRLF characters.
Use secure/httpOnly cookies.
When the mobile terminal uses OTP authentication, when calling generate OTP or Resend OTP API, OTP (One Time Password) cannot be returned directly. (Usually it is by sending a mobile phone to verify SMS, email random code and other methods, rather than direct response)
Limit the number of calls to APIs such as Login, Verify OTP, Resend OTP, and generate OTP for a single user, and use Captcha and other means to prevent brute force cracking.
Check the password reset token in the email or SMS to ensure randomness (cannot be guessed)
Set an expiration time for the token that resets the password.
After successfully resetting the password, the token used for resetting will become invalid.
User data and permission verification
For resource access such as my shopping cart and my browsing history, it is necessary to check whether the currently logged-in user has access rights to these resources.
To avoid continuous traversal of the resource ID, use /me/orders instead of /user/37153/orders in case you forget to check the permissions and cause data leakage.
To modify the email/phone number function, the user must first confirm that the user has verified that the email/phone is his own.
Any upload function should filter the file names uploaded by users. In addition, for universal reasons (not security issues), the uploaded things should be stored in cloud storage such as S3 (processed by lambda) instead of stored in Own server to prevent code execution. The personal avatar upload function should filter all EXIF tags, even if there is no such requirement.
User ID or other ID, should use RFC compliant UUID instead of integer. You can find the implementation of your language from github. JWT (JSON Web Token) is great. Use it when you need to build a single page application/API.
Android and iOS APP
The salt of the payment gateway should not be hardcoded
Secrets and auth tokens from third parties should not be hardcoded
APIs called between servers should not be called in the app
Under the Android system, carefully evaluate all requested permissions
In the iOS system, use the keychain of the system to store sensitive information (permission token, api key, etc.). Do not store this type of information in the user configuration.
Strongly recommend certificate pinning (Certificate pinning)
operating
If your business is small or you lack experience, you can evaluate using AWS or a PaaS platform to run code
Use formal scripts to create virtual machines on the cloud
Check all ports that are not necessarily open
Check whether the database has not set a password or use the default password, especially MongoDB and Redis
Use SSH to log in to your machine, do not use a password, but log in through SSH key authentication
Update the system in time to prevent 0day vulnerabilities, such as Heartbleed, Shellshock, etc.
Modify the server configuration, HTTPS uses TLS1.2, and disable other modes. (Worth it)
Do not enable DEBUG mode online, some frameworks, DEBUG mode will open many permissions and backdoors, or expose some sensitive data to the error stack information
Be prepared for bad guys and DDOS attacks, and use host services that provide DDOS cleaning
Monitor your system and record it in the log (for example, use New Relic or others).
If it is a 2B business, stick to the demand. If you use AWS S3, you can consider using the data encryption function. If you use AWS EC2, consider using the disk encryption function (now the system startup disk can also be encrypted)
About people
Open a mail group (for example: security@coolcorp.io) and collection page to facilitate security researchers to submit vulnerabilities
Depending on your business, restrict user database access
Be polite to people reporting bugs and vulnerabilities
Give your code to those who have a safe coding concept to review (More eyes)
In case of hacking or data leakage, check the log before data access and notify the user to change the password. You may need an external agency to help with the audit
Use Netflix Scumblr to keep abreast of some discussion information of your organization (company) on social networks or search engines, such as hacker attacks, vulnerabilities, etc.